Quick Examples
1D-vector
Find peaks in low sampled dataset
# Load library
from findpeaks import findpeaks
# Data
X = [9,60,377,985,1153,672,501,1068,1110,574,135,23,3,47,252,812,1182,741,263,33]
# Initialize
fp = findpeaks(lookahead=1)
results = fp.fit(X)
# Plot
fp.plot()
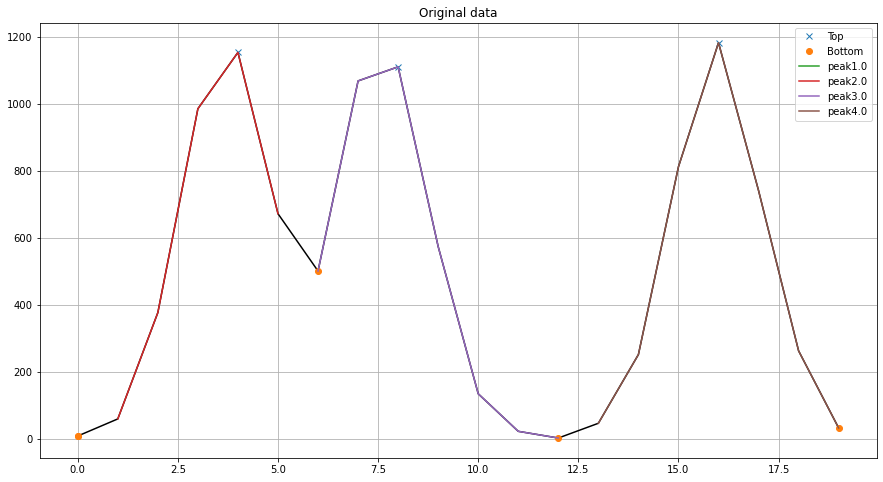
Interpolation
# Initialize with interpolation parameter
fp = findpeaks(lookahead=1, interpolate=10)
results = fp.fit(X)
fp.plot()
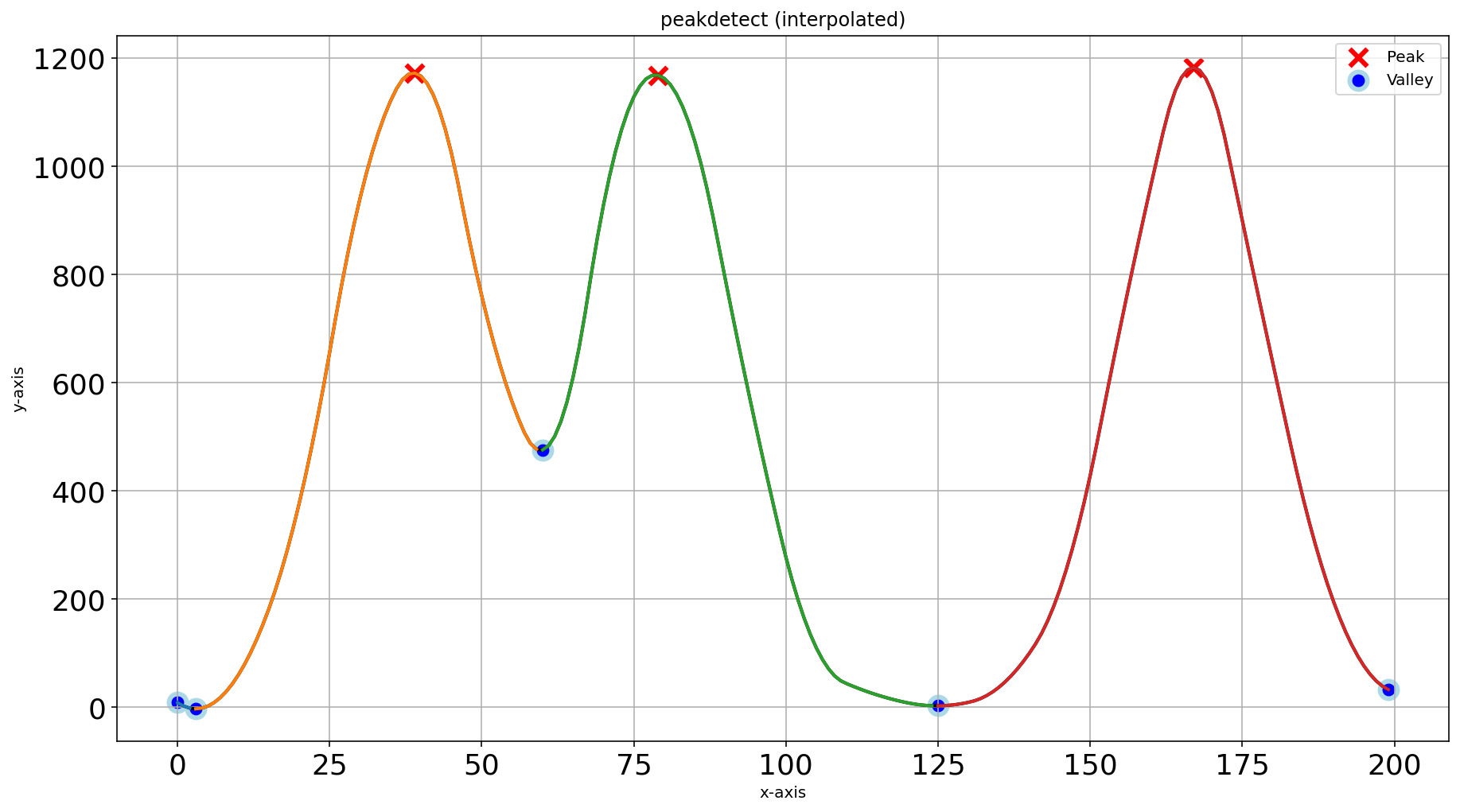
Comparison peak detection methods (1)
# Load library
from findpeaks import findpeaks
# Data
X = [10,11,9,23,21,11,45,20,11,12]
# Initialize
fp = findpeaks(method='peakdetect', lookahead=1)
results = fp.fit(X)
# Plot
fp.plot()
fp = findpeaks(method='topology', lookahead=1)
results = fp.fit(X)
fp.plot()
fp.plot_persistence()
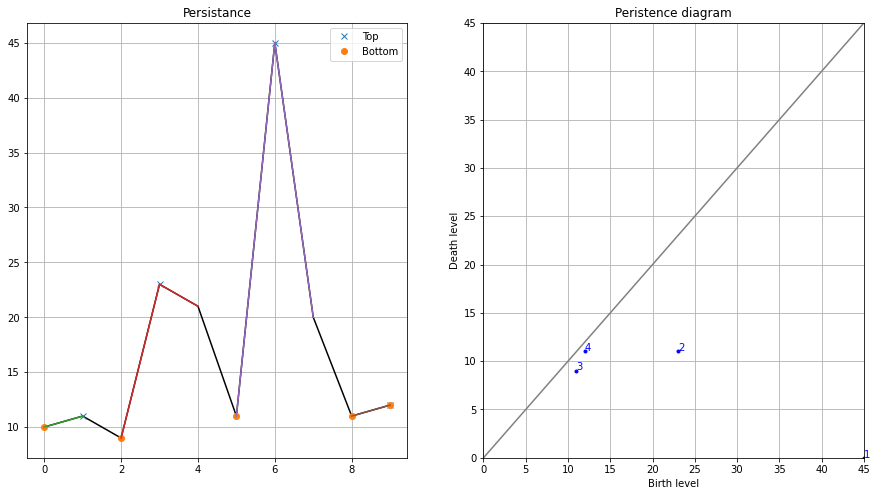
Comparison peak detection methods (2)
# Initialize with interpolate parameter
fp = findpeaks(method='peakdetect', lookahead=1, interpolate=10)
results = fp.fit(X)
fp.plot()
fp = findpeaks(method='topology', lookahead=1, interpolate=10)
results = fp.fit(X)
fp.plot()
Find peaks in high sampled dataset
# Load library
import numpy as np
from findpeaks import findpeaks
# Data
i = 10000
xs = np.linspace(0,3.7*np.pi,i)
X = (0.3*np.sin(xs) + np.sin(1.3 * xs) + 0.9 * np.sin(4.2 * xs) + 0.06 * np.random.randn(i))
# Initialize
fp = findpeaks(method='peakdetect')
results = fp.fit(X)
# Plot
fp.plot1d()
fp = findpeaks(method='topology', limit=1)
results = fp.fit(X)
fp.plot1d()
fp.plot_persistence()
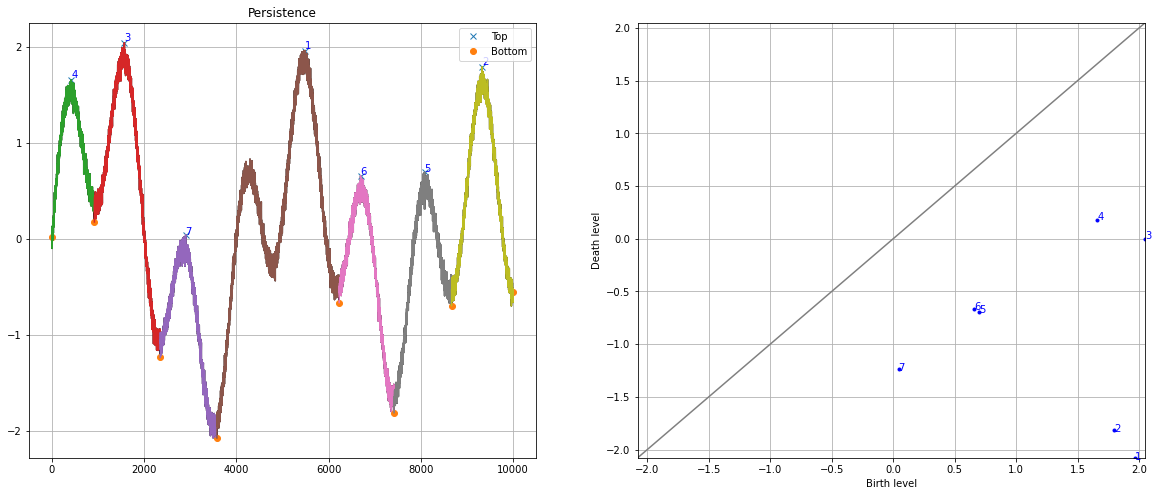
2D-array (image)
Find peaks using default settings
The input image:
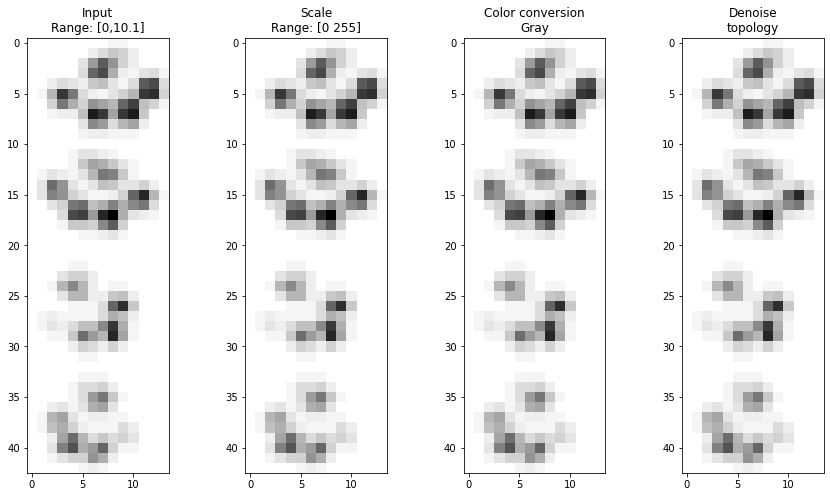
# Import library
from findpeaks import findpeaks
# Import example
X = fp.import_example()
print(X)
# array([[0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.4, 0.4],
# [0. , 0. , 0. , 0. , 0. , 0. , 0.7, 1.4, 2.2, 1.8],
# [0. , 0. , 0. , 0. , 0. , 1.1, 4. , 6.5, 4.3, 1.8],
# [0. , 0. , 0. , 0. , 0. , 1.4, 6.1, 7.2, 3.2, 0.7],
# [..., ..., ..., ..., ..., ..., ..., ..., ..., ...],
# [0. , 0.4, 2.9, 7.9, 5.4, 1.4, 0.7, 0.4, 1.1, 1.8],
# [0. , 0. , 1.8, 5.4, 3.2, 1.8, 4.3, 3.6, 2.9, 6.1],
# [0. , 0. , 0.4, 0.7, 0.7, 2.5, 9. , 7.9, 3.6, 7.9],
# [0. , 0. , 0. , 0. , 0. , 1.1, 4.7, 4. , 1.4, 2.9],
# [0. , 0. , 0. , 0. , 0. , 0.4, 0.7, 0.7, 0.4, 0.4]])
# Initialize
fp = findpeaks(method='mask')
# Fit
fp.fit(X)
# Plot the pre-processing steps
fp.plot_preprocessing()
# Plot all
fp.plot()
# Initialize
fp = findpeaks(method='topology')
# Fit
fp.fit(X)
The masking approach detects the correct peaks.
fp.plot()
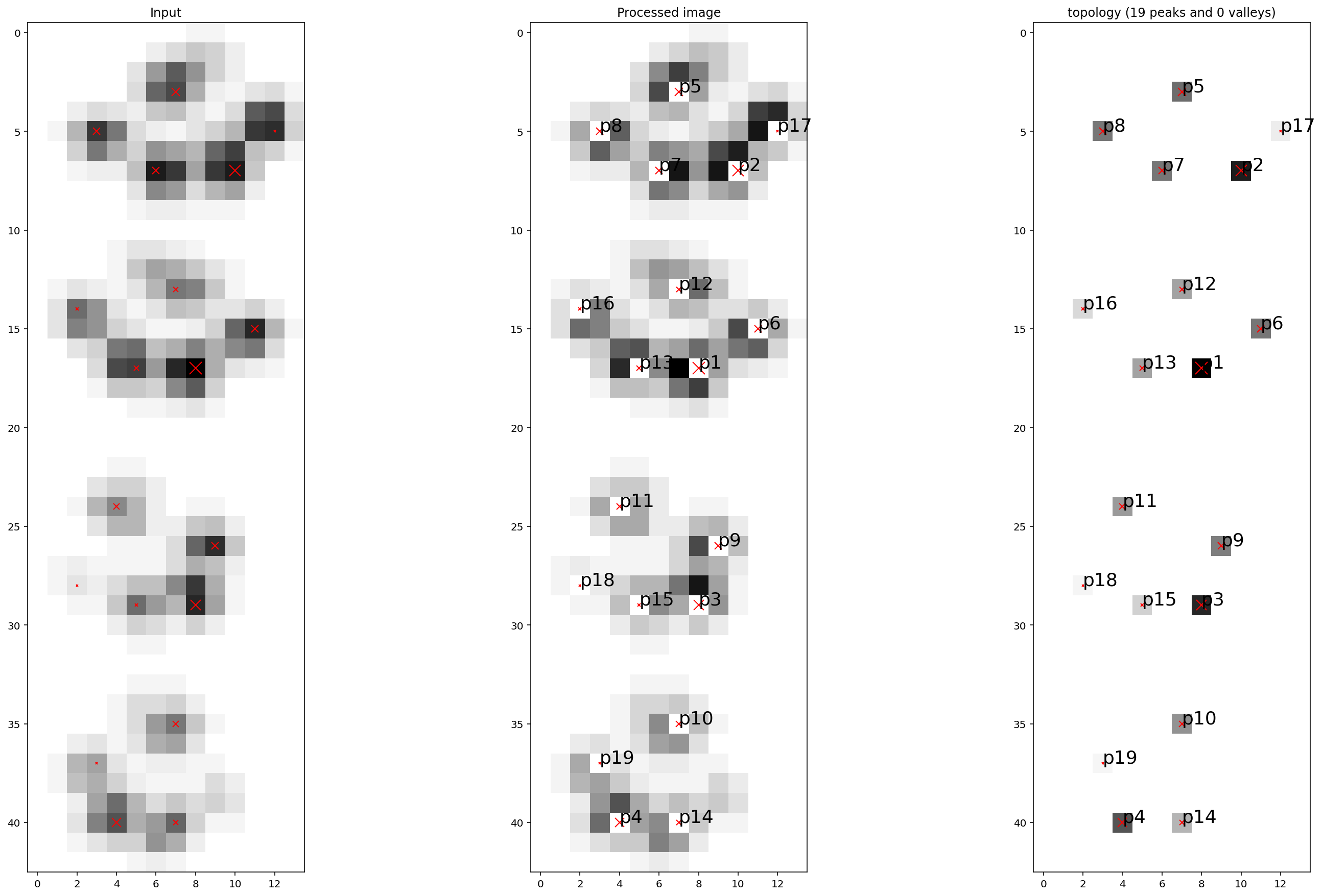
Conversion from 2d to 3d mesh plots looks very nice. But there is a rough surface because of the low-resolution input data.
fp.plot_mesh()
The persistence plot appears to detect the right peaks.
fp.plot_persistence()
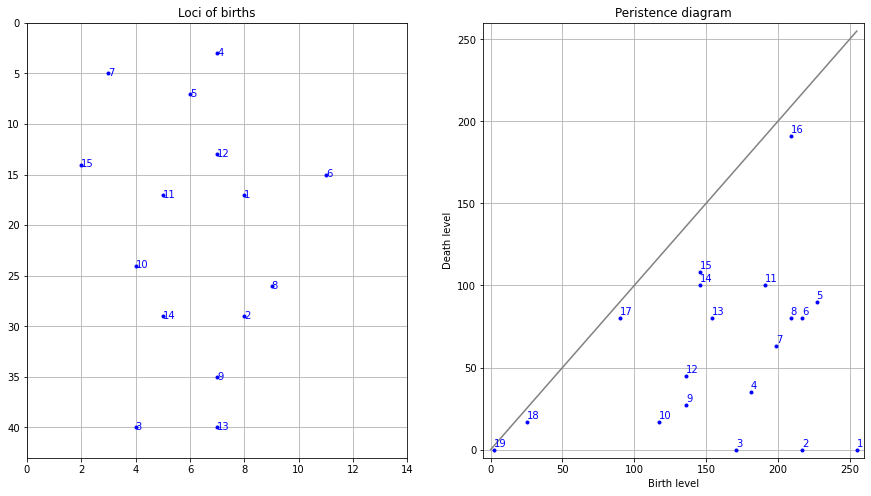
Find peaks with pre-processing
# Import library
from findpeaks import findpeaks
# Import example
X = fp.import_example()
# Initialize
fp = findpeaks(method='topology', scale=True, denoise=10, togray=True, imsize=(50,100), verbose=3)
# Fit
results = fp.fit(X)
# Plot all
fp.plot()
# Plot preprocessing
fp.plot_preprocessing()
The masking does not work so well because the pre-processing steps includes some weighted smoothing which is not ideal for the masking approach.
fp.plot()
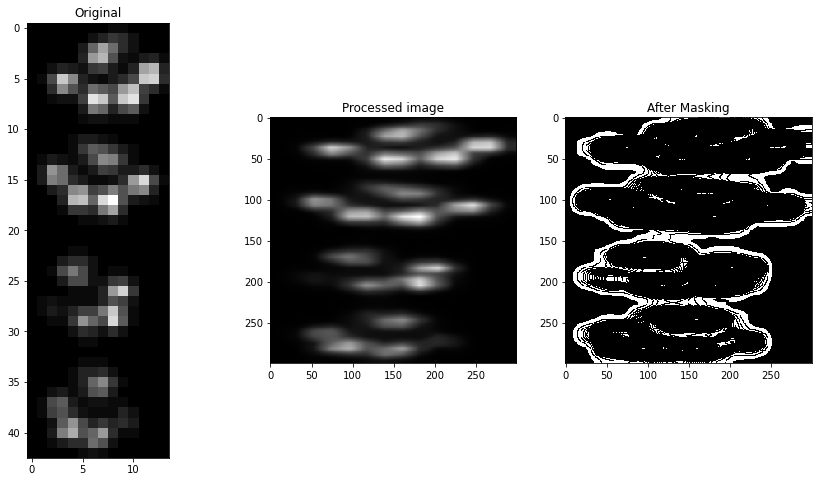
The mesh plot has higher resolution because the pre-processing steps caused some smoothing.
fp.plot_mesh()
The Persistence plot does show the detection of correct peaks.
fp.plot_persistence()
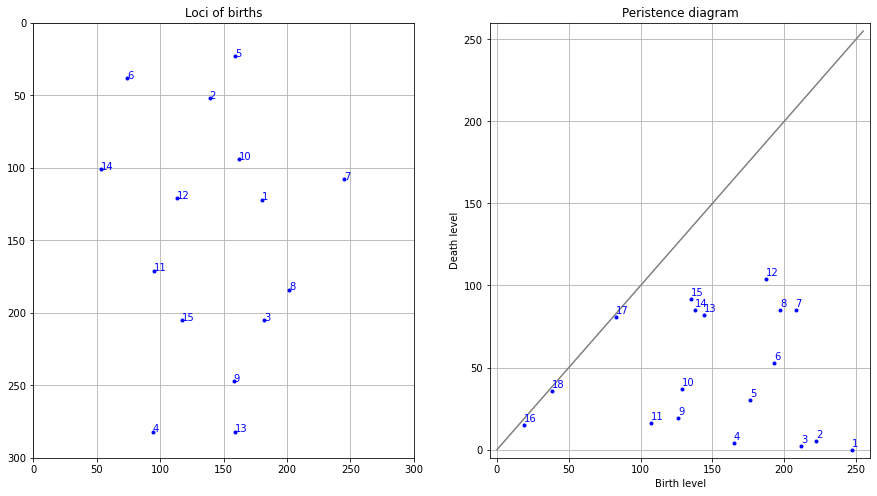