Generate N random colors
For the creation of N unique colors can be done with colourmap.colourmap.generate()
.
# Import library
import colourmap as colourmap
# Number of colors to print
N = 5
# Generate colors
c = colourmap.generate(N)
# Generate colors using different cmap
c_rgb = colourmap.generate(N, cmap='Set2')
print(c_rgb)
# array([[0.4, 0.76078431, 0.64705882],
# [0.55294118, 0.62745098, 0.79607843],
# [0.65098039, 0.84705882, 0.32941176],
# [0.89803922, 0.76862745, 0.58039216],
# [0.70196078, 0.70196078, 0.70196078]])
Create color based on input labels
For the creation of N unique colors for the N input labels can be performed with colourmap.colourmap.fromlist()
.
# Import library
import colourmap as colourmap
# Target labels
y=[1, 1, 2, 2, 3]
# String as input labels
y=["1", "1", "2", "2", "3"]
# Generate colors
c_rgb, c_dict = colourmap.fromlist(y)
print(c_rgb)
# [[0.89411765 0.10196078 0.10980392]
# [0.89411765 0.10196078 0.10980392]
# [1. 0.49803922 0. ]
# [1. 0.49803922 0. ]
# [0.6 0.6 0.6 ]]
print(c_dict)
# {1: array([0.89411765, 0.10196078, 0.10980392]),
# 2: array([1. , 0.49803922, 0. ]),
# 3: array([0.6, 0.6, 0.6])}
# Grab color for label 2
c_dict.get(2)
Color generated by seaborn and matplotlib
Two methods are implemented to generate the colormaps, with the function: colourmap.colourmap.generate()
.
seaborn
matplotlib
# Import library
import colourmap as colourmap
# Seaborn
c_rgb_1 = colourmap.generate(5, method='seaborn')
# Matplotlib
c_rgb_2 = colourmap.generate(5, method='matplotlib')
Convert RGB to HEX
Converting RGB to HEX can be performed with the function colourmap.colourmap.rgb2hex()
.
# Import library
import colourmap as colourmap
# String as input labels
y=["1", "1", "2", "2", "3"]
# Generate colors
c_rgb, c_dict = colourmap.fromlist(y)
print(c_rgb)
# [[0.89411765 0.10196078 0.10980392]
# [0.89411765 0.10196078 0.10980392]
# [1. 0.49803922 0. ]
# [1. 0.49803922 0. ]
# [0.6 0.6 0.6 ]]
# Convert to HEX
c_hex = colourmap.rgb2hex(c_rgb)
# Convert to HEX but keep alpha transparancy
c_hex = colourmap.rgb2hex(c_rgb, keep_alpha=True)
print(c_hex)
# array(['#e41a1c', '#e41a1c', '#ff7f00', '#ff7f00', '#999999'], dtype='<U7')
Convert HEX to RGB
Converting HEX to RGB can be performed with the function: colourmap.colourmap.hex2rgb()
.
# Import library
import colourmap as colourmap
print numpy as np
# String as input labels
y=["1", "1", "2", "2", "3"]
# Generate colors
c_rgb, c_dict = colourmap.fromlist(y)
print(c_rgb)
# [[0.89411765 0.10196078 0.10980392]
# [0.89411765 0.10196078 0.10980392]
# [1. 0.49803922 0. ]
# [1. 0.49803922 0. ]
# [0.6 0.6 0.6 ]]
# Convert to HEX
c_hex = colourmap.rgb2hex(c_rgb)
print(c_hex)
# array(['#e41a1c', '#e41a1c', '#ff7f00', '#ff7f00', '#999999'], dtype='<U7')
# Convert to HEX to RGB
c_rgb_1 = colourmap.hex2rgb(c_hex)
# Check
print(c_rgb_1)
# [[0.89411765 0.10196078 0.10980392]
# [0.89411765 0.10196078 0.10980392]
# [1. 0.49803922 0. ]
# [1. 0.49803922 0. ]
# [0.6 0.6 0.6 ]]
Linear gradient between two colors
A linear gradient can be created between two input colors with the function: colourmap.colourmap.linear_gradient()
.
# Import library
import colourmap as colourmap
# Create the gradient
colors = colourmap.linear_gradient("#000000", finish_hex="#FFFFFF", n=10)
# {'hex': ['#000000',
# '#1c1c1c',
# '#383838',
# '#555555',
# '#717171',
# '#8d8d8d',
# '#aaaaaa',
# '#c6c6c6',
# '#e2e2e2',
# '#ffffff'],
# 'r': [0, 28, 56, 85, 113, 141, 170, 198, 226, 255],
# 'g': [0, 28, 56, 85, 113, 141, 170, 198, 226, 255],
# 'b': [0, 28, 56, 85, 113, 141, 170, 198, 226, 255]}
Linear gradient based on input labels
A linear gradient can be created based on the input labels with the function: colourmap.colourmap.fromlist()
.
The input labels are used to create unique colors based on
cmap
.A gradient is computed starting with each unique color towards the defined color in
gradient
.The most dense regions is the start of the gradient and will linear transform towards the edges.
# Import library
import colourmap as colourmap
# Example dataset
import matplotlib.pyplot as plt
from sklearn import datasets
iris = datasets.load_iris()
X = iris.data[:, :2]
labels = iris.target
# Gradient per class label
label_colors, colordict = colourmap.fromlist(labels, cmap='Set1', gradient='#FFFFFF')
# Scatter with gradient per class label
plt.figure(figsize=(15,10))
plt.scatter(X[:,0],X[:,1], c=label_colors)
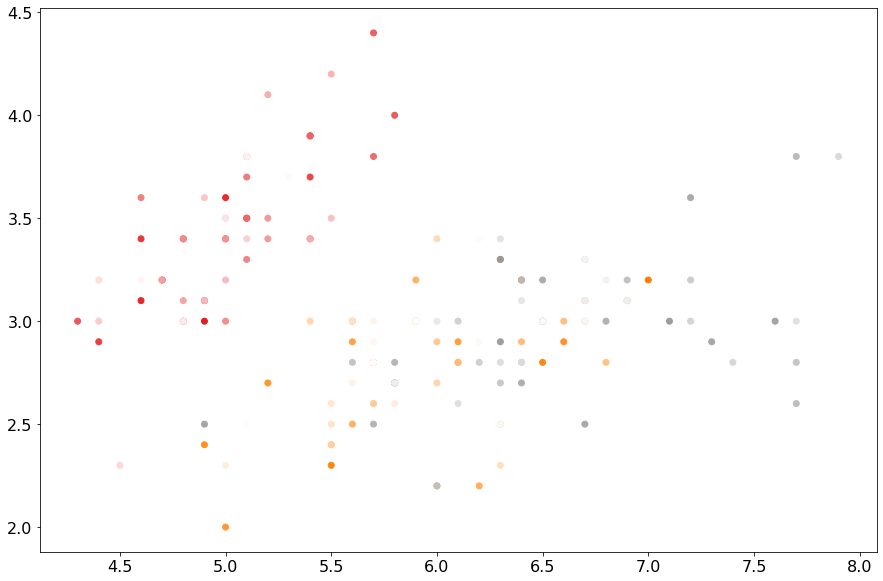